In a project like this, implementing a simple enemy AI is surprisingly easy. This is because your player largely already runs off of an AI system. The player uses their input to decide when and where to move and use abilities, but things like pathing, range checking, even aiming to some degree, are done by the player character's AI. Because of this, making an enemy with functioning AI is largely just a matter of creating another player character with a way to detect and select its own targets and trigger its own ability inputs. This could of course be made more advanced later by having enemies behave more intelligently when selecting targets, positions and ability timings, but for now this is not needed. All we need at the moment is an enemy which can do what the player does; see their opponent, get into range, and use their abilities on them until somebody dies.
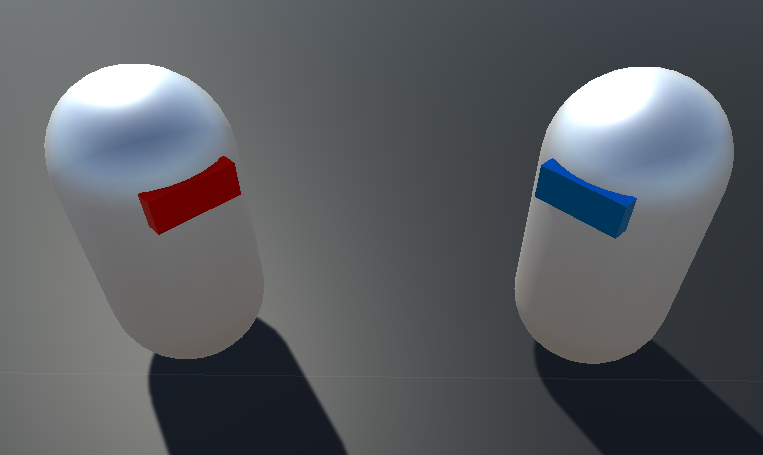
To begin, I created a dummy enemy, seen in my last post. This dummy was pretty identical to the player except it has a enemy controller script rather than a player controller. This also inherits from the base character script though so it has all the same variables except for the references to the camera and UI. To allow the enemy to detect a player coming into range, I gave it an additional collider, a sphere, set as a trigger. I then added a game object list variable and wrote the following code to track players in the enemy's detection range.
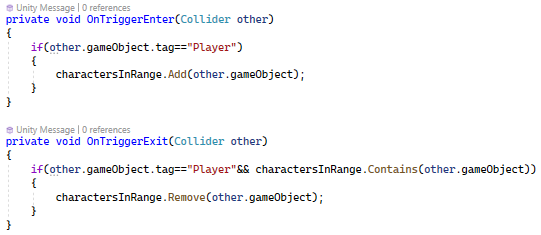
I then added the following code to the update function which sets a player as the target, set the enemy as in combat, and calls a function to try and use abilities on that target.
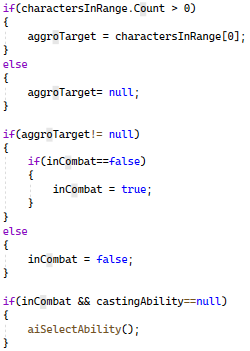
The aiSelectAbility function then picks a random ability and tries to cast it if it is not on cooldown.

Over in the ability script, we detect if the character using the ability is an enemy and call the corresponding targeting function.
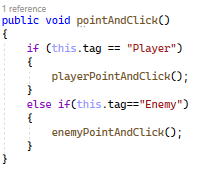
The enemy point and click function then attempts apply the abilities effects to the target if they are in range. If not, it calls the move into range function, causing the enemy to run closer to their target.

The move into range and apply effect functions are shared between the player and enemy controller so there is nothing new there. As explained earlier, these characters behave similarly, the only real difference is whether they use player or AI input. Now that we have multiple characters moving around, simply setting the nearest in range location as the destination when casting an ability will not work as the target may have moved by the time you get there. To solve this I added lines to the update function which continually edits this in-range destination until the character successfully casts the ability or, in the case of a player, uses another move or ability input. This cancelling of the chase functionality works because the activate ability and move functions set the target character and casting ability to null before selecting a new target.

Here is a video of the enemy AI detecting the player, running into range, and damaging them with an ability, then doing so again once their ability is off cooldown:
Comments