Previously, I went through the implementation of your fist multi-target ability type, a piercing projectile. This can allow characters to take out multiple opponents in a line, but we want to take things further and allow you to take down whole groups of enemies in one go. To accomplish this, I have implemented AoE (area of effect) abilities. More specifically I have implemented circular, ground-targeted AoEs. Other variations such as directional cones may be implemented later.
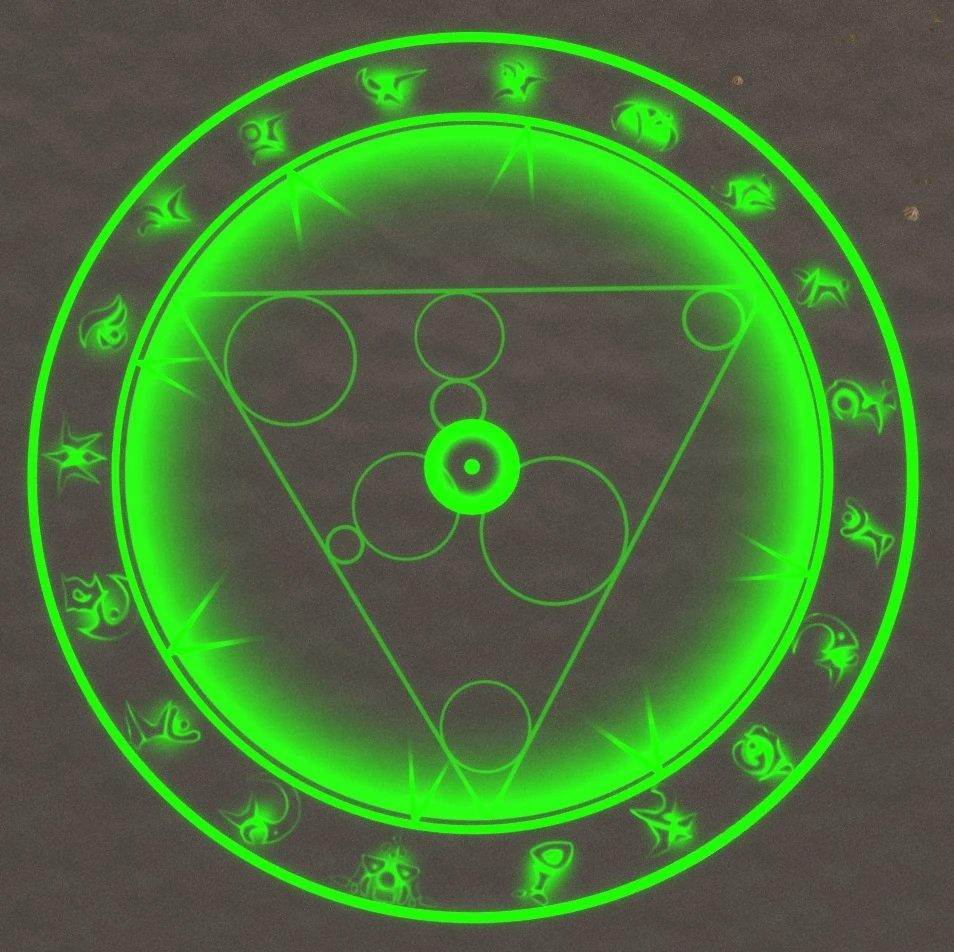
Before we got onto that though, I'll go through an additional bit of functionality I made to the effects system, stacking. A "stacking" effect is one that can be applied multiple times to the same target with each application (known as a stack) adding together to form a stronger effect. For example, if a DoT is stacking and deals 1 dps (damage per second) and you apply a second stack of the DoT, the target will now be taking 2 dps. In some cases, this will also refresh the duration of the effect but for now that is not what we will be doing. If an effect does not stack, some games may have a re-application have no effect. In this project however, attempting to apply a non-stacking effect to an already affected target, will refresh the duration of the existing effect. Previously, all effects in this game stacked as you could not repeatedly apply them due to ability cooldowns. If you got your cooldowns low enough and duration high enough to stack an effect then that would be a fun bonus, but it wouldn't come up too often. With the implementation of AoEs however, it was necessary to make some effects non-stacking as I want some AoEs to simply apply effects to targets and others to continuously apply a stacking effect the longer the target remains in the area. This should allow more gameplay variation for those interested in an effect-based playstyle.
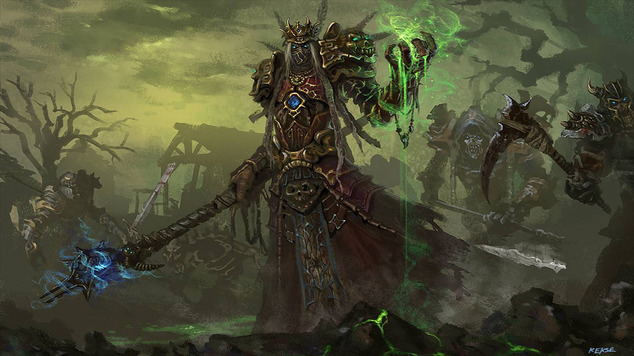
To begin, I added a bool called stackingEffect to the base ability script. I then added lots of additional functionality to the create effect function. If stacking effect is true, then nothing new happens, when create effect is called, it adds the effect to the target. If stacking effect is false then we create a local "alreadyApplied" bool and check through the target's child objects to see if any are effects created by this ability. If a matching effect is found, we refresh the duration and set already applied to true. If no matching effects are found, already applied remains false and we apply the effect.
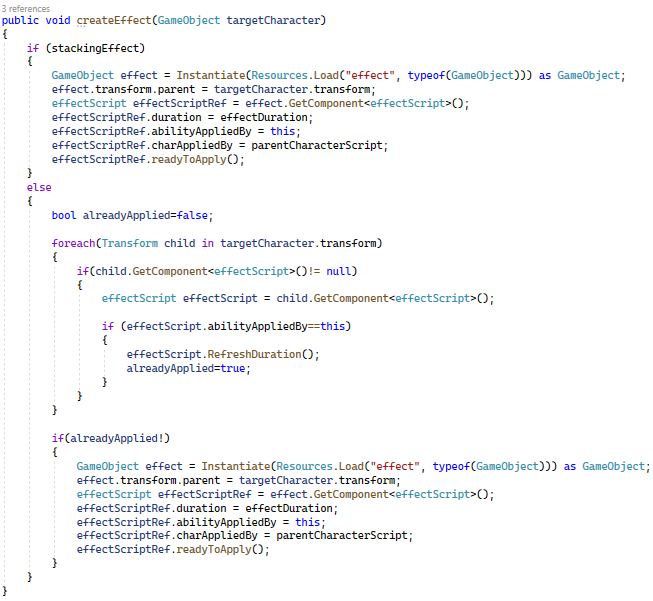
In the effect script, I also added a short function to refresh the effect's duration when a non-stacking effect is re-applied.
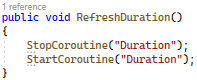
Now lets move onto the AoEs. I began by adding an AoE duration and "offensive" bool to the ability script. AoE duration, of course, will determine how long the AoE will persist after the ability is cast. An explosion may only last a moment, dealing a single instance of damage. A poisonous cloud may last a while, constantly damaging enemies inside. The offensive bool determines if an ability is meant to help or hinder. If an ability is offensive, it will only effect the user's opponents, if it is not, it will only effect their allies. This will become important when groups of enemies are in combat or when the player is able to summon minions. I added this now because this is our first ability type that is very likely to hit things other than the primary target.

In the activate ability function, another option has been added to a new "groundTarget" function if the ability's targeting is set to "ground".
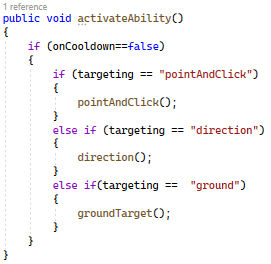
If used by a player character, the ground target effect will attempt to spawn the AoE at their mouse location if in range, or will do so after walking into range if too far away. An enemy will instead attempt to spawn the AoE on top of their target character, making sure to move into range first if too far away.
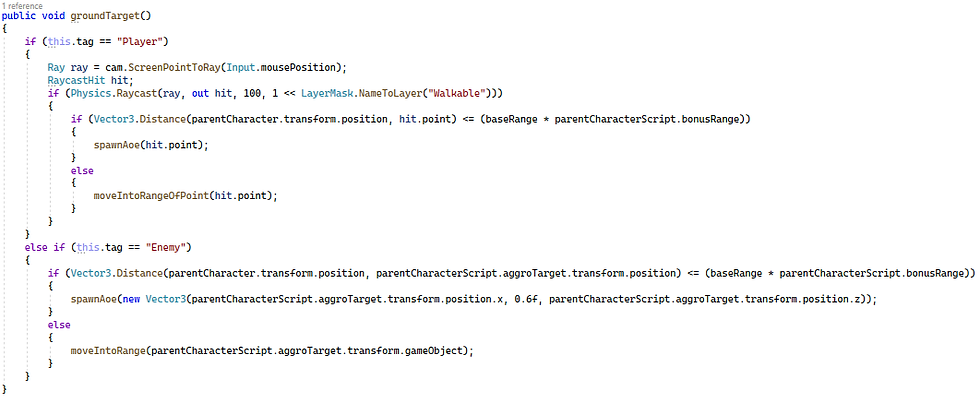
Since the move into range function takes a game object input and the player is not targeting a game object here, i created a move into range of point function and added a vector 3 bool called target position to the base character. This allows the player to set their destination in relation to the ground position rather than a target character's position.

Over in the update function of the player controller, a check was added to trigger the spawn AoE function once in range of the target position.

The spawn AoE function turns the character to the AoE spawn point, instantiates the AoE prefab at that point with no rotation since it is circular. The X and Z scale are then set based on the ability's base AoE radius value and the character's bonus area stat. The Y value is set to 0.1 as additional height will no increase the size of the effected area in-game since we are playing on a flat surface, but will obscure vision more. We then pass over which character and ability spawned the AoE, set its damage and duration and if it is offensive or not, before calling the ready to activate function in the AoE and starting the ability's cooldown.
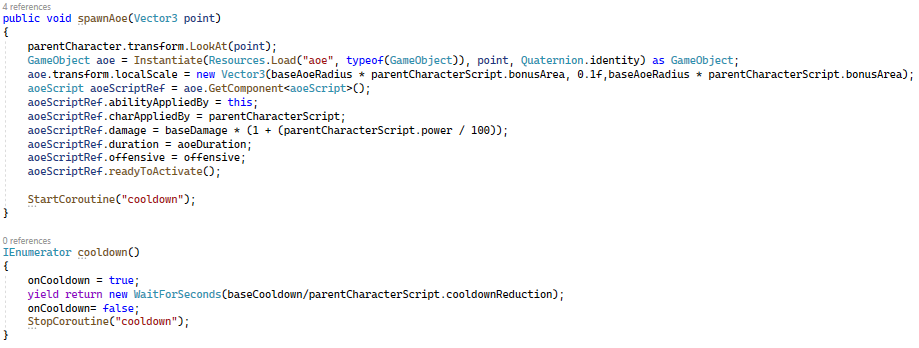
Now we have all the functionality to spawn the AoE, lets build the prefab we are trying to spawn. I created a cylinder in the scene, made it very thin, raised it just above ground level, set its collider to trigger, gave it a rigidbody with locked rotation and no gravity, and created an AoE script which I attached. I also make a placeholder transparent green mesh to differentiate it from over things. This object was then pulled into the resources folder to make it a loadable prefab and then it was deleted from the scene.
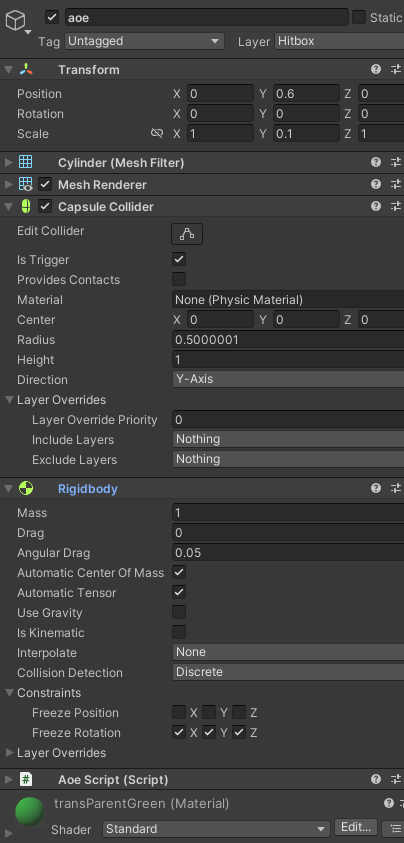
The AoE script begins by declaring the variables we set in the spawn AoE function.
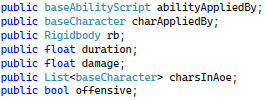
Then we have the ready to activate function which is called by the ability script once the variables are set. This calls the apply effects function, making the AoE functional. Note that the apply effects function here is a coroutine rather than a function, this is so that it can feature delays, allowing it to be repeated at a set rate.

Next up are the on trigger enter and exit functions. If the AoE is offensive, these add opponent's within the AoE to its list of targets. If it is non-offensive, it only adds allies to the list. Either way, if a target exits the AoE, they are removed from the list.
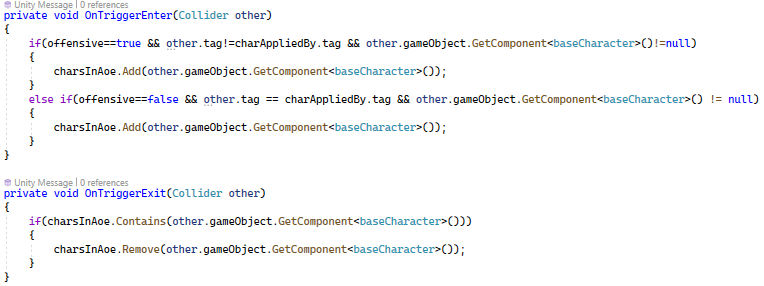
When apply effects is called, it will apply the damage and effects of the ability that spawned it to all viable targets within its collider. The duration timer coroutine is then started. The duration timer waits for the duration to complete and then destroys the AoE. If we want this to just be an instant AoE ability like an explosion, we can set the duration timer to be equal to or less then the wait for seconds in the apply effect coroutine. This will mean that the duration will expire and destroy the AoE after the effects are applied once but before apply effect repeats itself. If we want the AoE to linger and continuously apply effects to targets within it, we can set the duration to be longer. The apply effects coroutine has a fixed delay of 0.1 seconds to keep it in line with the tick-rate of DoTs. This means it will constantly apply damage or effect stacks at a fixed rate while the target is within the AoE.
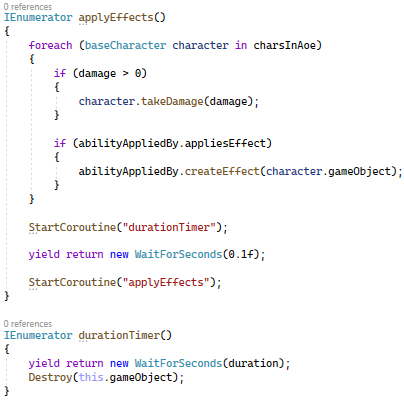
Here is a video of some AoE abilities in action:
Comments